Overview
This addition will allow users to see the history of directives made to a column of data.
Goals
User should be able to see lineage information, ie. directives, for columns
Storing lineage information should have minimal/no impact to the wrangler application
User Stories
As a user, I should be able to see the directives applied to a column of data.
As a user, I should be able to see the directives applied to a column of data over any period of time.
- As a user, I should be able to see how any column got to its current state as well as other columns that were impacted by it
As a user, I should be able to add tags and properties to specific columns of data (stretch)
Design
Save directives for each column in AST format after parsing of directives along with necessary information (time, dataset/stream name/id, etc.).
prepareRun() api to send information to platform.
Unmarshal and connect into graph and store in HBase.
Access to lineage should only be available through the platform
Questions
- Does ParseTree have all necessary information for every directive?
Approach
Computing Lineage Approach:
Compute lineage without looking at data by backtracking
Advantages:
- No instance variables added to step classes
- Faster
Disadvantages:
- Requires stricter rule on directives, ie. every rename must give old and new name. See * below for why
*Backtrack starting with columns A,B,C. Previous directive is "set-columns A B C". The directive before that is "lowercase <column>" where <column> is nameOfOwner. No way of knowing what nameOfOwner refers to without looking at data.
API changes
New Programmatic APIs:
TransformStep:
TransformStep is a Java interface that represents a modification done to a dataset by a transform stage.
import com.sun.istack.internal.Nullable;
/**
* <p>A TransformStep represents a modification done by a Transform.</p>
*/
public interface TransformStep {
/**
* @return the name of this modification
*/
String getName();
/**
* @return additional information about this modification
*/
@Nullable
String getInformation();
}
FieldLevelLineage:
FieldLevelLineage is a Java interface that contains all the necessary field-level lineage information to be sent to the CDAP platform, for transform stages.
import java.util.List;
import java.util.Map;
/**
* <p>FieldLevelLineage is a DataType for computing lineage for each field in a dataset.
* An instance of this type can be sent to be sent to platform through API for transform stages.</p>
*/
public interface FieldLevelLineage {
/**
* <p>A BranchingTransformStepNode represents a linking between a {@link TransformStep} and a field of data.
* It contains data about how this TransformStep affected this field.</p>
*/
interface BranchingTransformStepNode {
/**
* @return the index of the TransformStep in {@link #getSteps()}
*/
int getTransformStepNumber();
/**
* @return true if this TransformStep does not add this field
*/
boolean continueBackward(); // continue down
/**
* @return true if this TransformStep does not drop this field
*/
boolean continueForward(); // continue up
/**
* This map should contain every other field that was impacted by this field in this TransformStep
* @return A map from field name to the index of the next TransformStep using {@link #getLineage()}
* Usage: getLineage().get(field).get(index).
*/
Map<String, Integer> getImpactedBranches(); // getUpBranches
/**
* This map should contain every other field that impacted this field with this TransformStep
* @return A map from field name to the index of the previous transformation step in {@link #getLineage()}
* Usage: getLineage().get(field).get(index).
*/
Map<String, Integer> getImpactingBranches(); // getDownBranches
}
/**
* @return a list of all TransformSteps executed by this Transform in order.
*/
List<TransformStep> getSteps();
/**
* @return a mapping of field names to a list of {@link BranchingTransformStepNode} in reverse order.
*/
Map<String, List<BranchingTransformStepNode>> getLineage();
}
WranglerFieldLevelLineage:
Instance should be initialized per wrangler node passing in a list of final columns (output schema) and the name of the wrangler node.
store() takes a ParseTree and stores all the necessary information into lineages.
Stores lineage for each column in lineage instance variable which is a map to ASTs.
import com.google.common.collect.BiMap;
import com.google.common.collect.HashBiMap;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* DataType for computing lineage for each column in Wrangler
* Instance of this type to be sent to platform through API
*/
public class WranglerFieldLevelLineage implements FieldLevelLineage {
private static final String PARSE_KEY = "all columns";
private enum Type {
ADD, DROP, MODIFY, READ, RENAME
}
public class WranglerBranchingStepNode implements BranchingTransformStepNode {
final int stepNumber;
boolean continueUp;
boolean continueDown;
Map<String, Integer> upBranches;
Map<String, Integer> downBranches;
WranglerBranchingStepNode(int stepNumber, boolean continueUp, boolean continueDown) {
this.stepNumber = stepNumber;
this.continueUp = continueUp;
this.continueDown = continueDown;
this.upBranches = new HashMap<>();
this.downBranches = new HashMap<>();
}
void putRead(String columnName) {
upBranches.put(columnName, lineage.get(columnName).size() - 1);
}
void putLineage(String columnName) {
downBranches.put(columnName, lineage.get(columnName).size());
}
@Override
public int getTransformStepNumber() {
return this.stepNumber;
}
@Override
public boolean continueBackward() {
return this.continueDown;
}
@Override
public boolean continueForward() {
return this.continueUp;
}
@Override
public Map<String, Integer> getImpactedBranches() {
return Collections.unmodifiableMap(this.upBranches);
}
@Override
public Map<String, Integer> getImpactingBranches() {
return Collections.unmodifiableMap(this.downBranches);
}
// toString()
}
private int currentStepNumber;
private final Set<String> currentColumns;
private final TransformStep[] steps;
private final Map<String, List<BranchingTransformStepNode>> lineage;
public WranglerFieldLevelLineage(int numberOfSteps, List<String> finalColumns) {
this.currentColumns = new HashSet<>(finalColumns.size());
this.steps = new WranglerTransformStep[numberOfSteps];
this.lineage = new HashMap<>(finalColumns.size());
for (String key : finalColumns) {
this.currentColumns.add(key);
this.lineage.put(key, new ArrayList<BranchingTransformStepNode>());
}
}
// store() helpers
public void store(WranglerStep[] parseTree) {...} // builds lineage data structure
@Override
public List<TransformStep> getSteps() {
return Collections.unmodifiableList(Arrays.asList(this.steps));
}
@Override
public Map<String, List<BranchingTransformStepNode>> getLineage() {
return Collections.unmodifiableMap(this.lineage);
}
// toString()
}
Parse Tree should contain all columns affected per directive.
Labels:
- All columns should be labeled one of: {Read, Drop, Modify, Add, Rename}
- Read: column's name or values are read. Including reading values and modifying. ie. "filter-rows-on..."
- Drop: column is dropped
- Add: column is added
- Rename: column's name is replaced with another name
- Modify: column's values altered and doesn't fit in any of the other categories, ie. "lowercase"
For Read, Drop, Modify, and Add the column and associated label should be something like -> Column: Name, Label: add.
For Rename the column and associated label should be something like -> Column: body_5 DOB, Label: rename. // Basically some way of having both names, currently using a space. Old/new for rename. Swap example: A B, Label: rename and B A, Label: rename. Both the old & new names should be part of the information being sent somehow.
For Read, Modify, and Add there is another option; instead of column name can return {"all columns", "all columns minus _ _ _ _ ", "all columns formatted %s_%d"}, along with label. ie. Column: "all columns minus body", Label: add. "all columns" refers to all columns present in dataset after execution of this step. Format string only accepts %s and %d.
For Rename, and Drop this option is not available; must explicitly return name of all columns involved.
**Assumption (can be changed): In ParseTree all columns should be in order of impact. ie. If directive is "copy A A_copy". "A, Label: read" should be before "A_copy, Label: add".
Algorithm visual: Example wrangler application --> lineage --> ASTs for each column
FieldLevelLineageStorageNode:
FieldLevelLineageStorageNode is a Java interface that represents an element being stored in HBase.
These nodes are created and connected in an AST format by FieldLevelLineageStorageGraph.
/**
* <p>A FieldLevelLineageStorageNode represents a node of field-level lineage information.</p>
*/
public interface FieldLevelLineageStorageNode {
/**
* @return the ID of the pipeline
*/
ProgramRunId getPipeline();
/**
* @return the name of the stage
*/
String getStage();
/**
* @return the name of the field
*/
String getField();
}
FieldLevelLineageStorageGraph:
FieldLevelLineageStorageGraph is a Java class that transforms many instances of FieldLevelLineage into a graph of FieldLevelLineageStorageNodes for storage.
One instance of this type should be created per pipeline. Created from many instances of FieldLevelLineage, some graph representation of the pipeline, a mapping between reference names and stage names for sources/sinks, and ProgramRunId.
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.HashBasedTable;
import com.google.common.collect.ListMultimap;
import com.google.common.collect.Table;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public final class FieldLevelLineageStorageGraph {
private final ProgramRunId pipelineId;
private final PipelinePhase pipeline; // Or another type of graph of the pipeline
private final Map<String, FieldLevelLineage> stages;
private final Map<String, String> stageToDataSet;
private final Map<FieldLevelLineageStorageNode, FieldLevelLineageStorageNode> nodeRetriever;
private final Table<String, String, DataSetFieldNode> history; // Storage
private final Map<FieldStepNode, TransformStep> stepInformation; // Storage
private final ListMultimap<FieldLevelLineageStorageNode, FieldLevelLineageStorageNode> pastEdges; // Storage
private final ListMultimap<FieldLevelLineageStorageNode, FieldLevelLineageStorageNode> futureEdges; // Storage
public FieldLevelLineageStorageGraph(ProgramRunId PipelineId, PipelinePhase pipeline,
Map<String, FieldLevelLineage> stages, Map<String, String> stageToDataSet) {
this.pipelineId = PipelineId;
this.pipeline = pipeline;
this.stages = stages;
this.stageToDataSet = stageToDataSet;
this.nodeRetriever = new HashMap<>();
this.history = HashBasedTable.create();
this.stepInformation = new HashMap<>();
this.pastEdges = ArrayListMultimap.create();
this.futureEdges = ArrayListMultimap.create();
}
// helpers for make()
private void make() {...} // makes the graph
}
Visual:
Publishing Lineage Information:
Will be done through new prepareRun() function in the Transform class and new recordLineage(FieldLevelLineage f) function in StageSubmitter interface. Each transform plugin stage will have option to publish field-level lineage.
Wrangler Example:
...
@Override
public void prepareRun(StageSubmitter<TransformContext> context) throws Exception {
List<String> endColumns = new ArrayList<>(oSchema.getFields().size());
for (Schema.Field field : oSchema.getFields()) {
endColumns.add(field.getName());
}
FieldLevelLineage f = new WranglerFieldLevelLineage(StringUtils.countMatches(config.directives, "\n"),
endColumns);
// ((WranglerFieldLevelLineage) f).store(this.parseTree);
context.recordLineage(f);
}
...
New REST APIs
Path | Method | Description | Response |
---|
/v3/namespaces/{namespace-id}/datasets/{dataset-id}/columns/{column-id}/lineage?start=<start-ts>&end=<end-ts>&maxLevels=<max-levels> | GET | Returns list of directives applied to the specified column in the specified dataset | 200: Successful Response TBD, but will contain a Tree representation |
/v3/namespaces/{namespace-id}/streams/{stream-id}/columns/{column-id}/lineage?start=<start-ts>&end=<end-ts>&maxLevels=<max-levels> | GET | Returns list of directives applied to the specified column in the specified stream | 200: Successful Response TBD, but will contain a Tree representation |
CLI Impact or Changes
TBD
UI Impact or Changes
- Option 2: Add interface to metadata table when viewing dataset to see lineage of columns possibly by clicking on column: -> When a column is clicked on will look something like:
-> 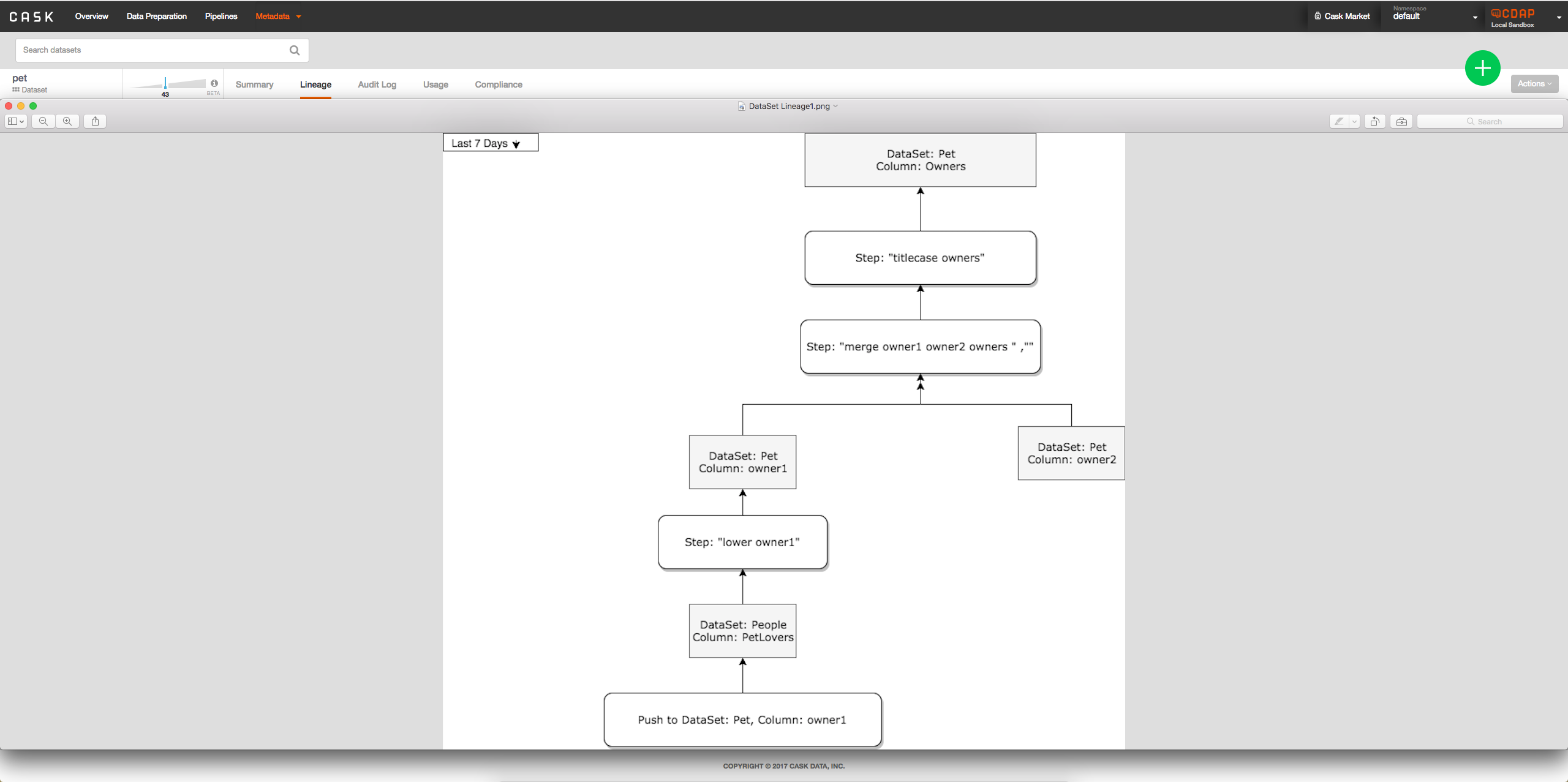
- Option 2: Show all columns at once directly on lineage tab from clicking on dataset, tab between field level and dataset level:
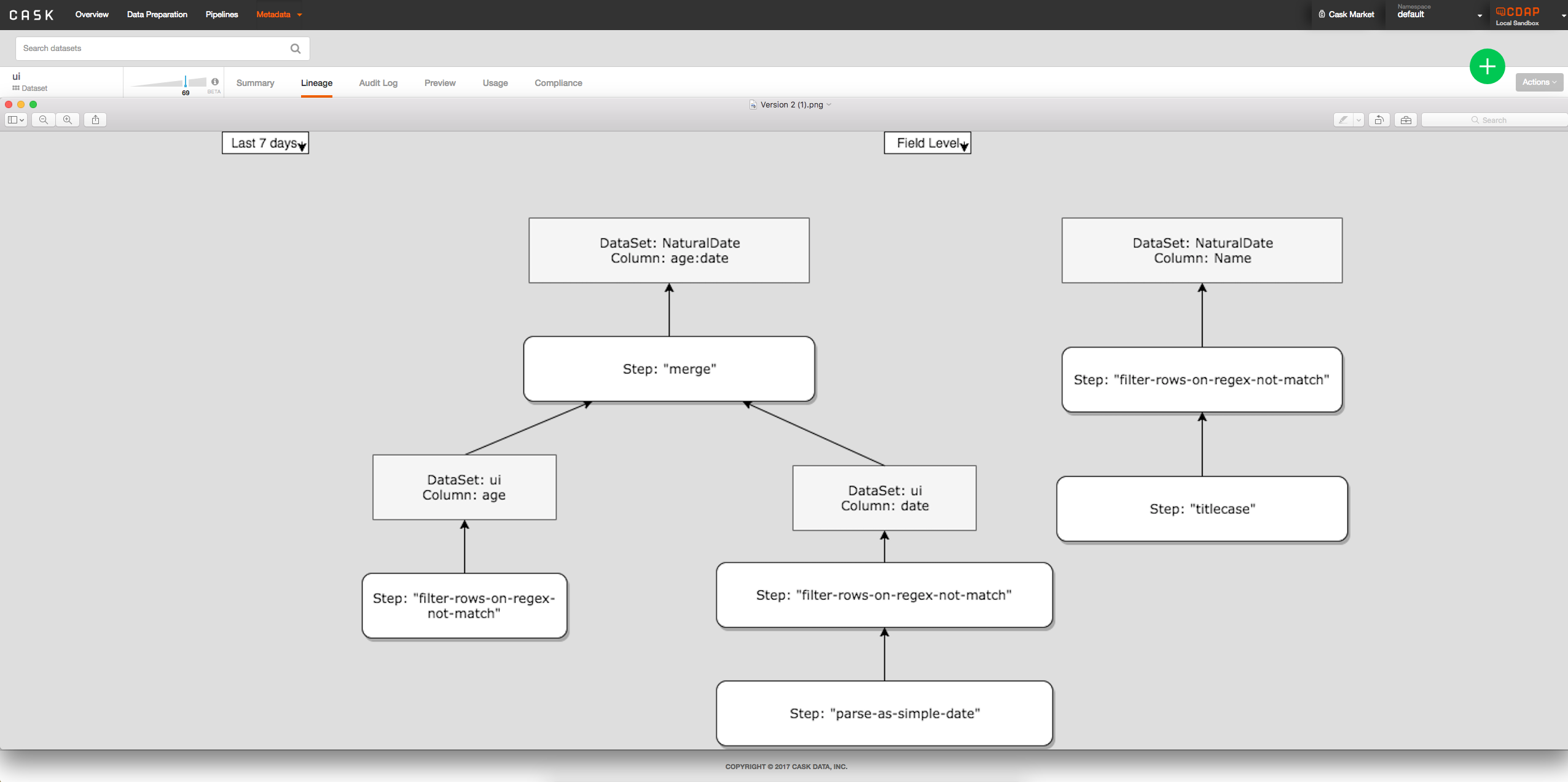
Security Impact
Should be none, TBD
Impact on Infrastructure Outages
Storage in HBase. Key being dataset reference name + field name + ProgramRunId. For each pipeline a node is added to this key; Impact TBD.
Test Scenarios
Test ID | Test Description | Expected Results |
---|
1 | Tests all directives | All Step subclasses should be properly parsed containing all correct columns with correct labels |
2 | Multiple datasets/streams | Lineages are correctly shown between different datasets/streams |
3 | Tests all store() | FieldLevelLineage.store() always correctly stores step |
Releases
Release 4.3.0
Release 4.4.0
- Fixing TextDirectives and parsing of directives in general